Before reading the post, if you need the VHDL code example of the Digital MUX, just put your email in the box you find in the post. There is no need to post a comment asking me for the code 🙂
If you don’t receive the email, please check your SPAM folder, enjoy!
What is a MUX?
When we implement a digital hardware architecture, we often need to select an input to our logic between several different inputs. This selection logic is called digital multiplexer or MUX.
We name it digital multiplexer, to distinguish it from an analog multiplexer. An analog multiplexer implements the same function as digital MUX selecting the source of a signal from different analog source instead of digital.
As clear in Figure1, a MUX can be visualized as an n-way virtual switch whose output can be connected to one of the different input sources. On the left side of the Figure1, you can see the typical MUX representation. The number near the input ports indicates the selector value used to route the selected input to the output port.
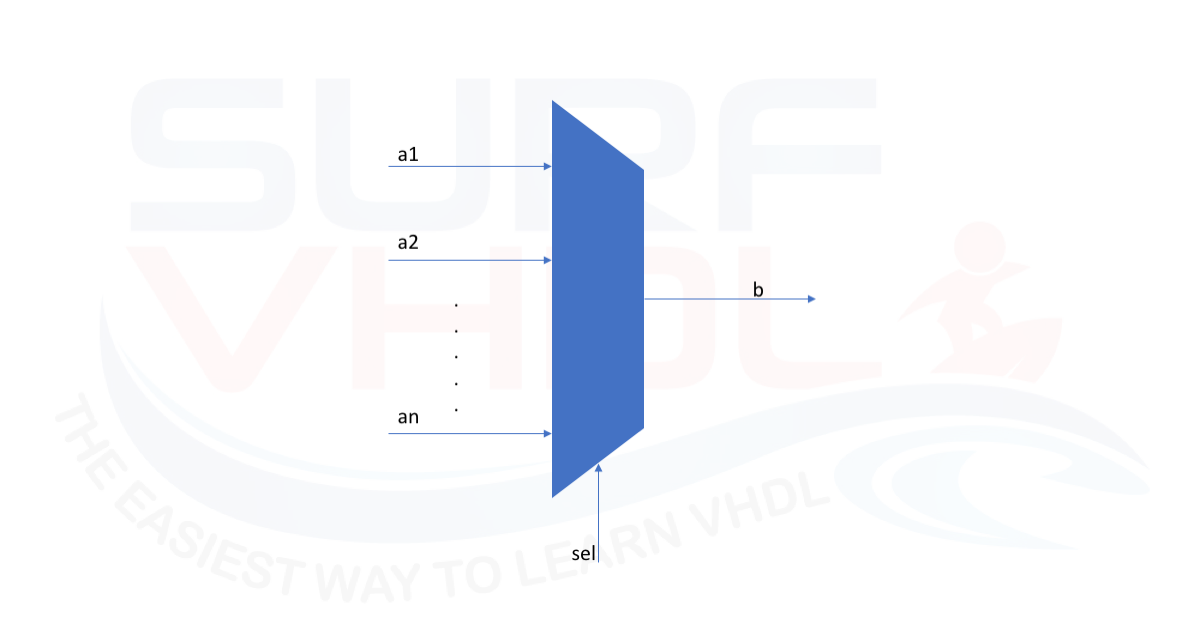
VHDL implementation of a digital MUX
The digital MUX is one of the basic building blocks of a digital design. Using the VHDL we have basically two different ways to describe a digital MUX:
- Concurrent description
- Sequential description
Both the descriptions are totally equivalent and implement the same hardware logic. You can use concurrent or sequential depending on your coding style.
Here below is represented a 4-way mux using a sequential representation
library IEEE; use IEEE.std_logic_1164.all; entity mux4 is port( a1 : in std_logic_vector(2 downto 0); a2 : in std_logic_vector(2 downto 0); a3 : in std_logic_vector(2 downto 0); a4 : in std_logic_vector(2 downto 0); sel : in std_logic_vector(1 downto 0); b : out std_logic_vector(2 downto 0)); end mux4; architecture rtl of mux4 is -- declarative part: empty begin p_mux : process(a1,a2,a3,a4,sel) begin case sel is when "00" => b <= a1 ; when "01" => b <= a2 ; when "10" => b <= a3 ; when others => b <= a4 ; end case; end process p_mux; end rtl;
MUX description using SEQUENTIAL VHDL statement
Here below is represented a 4-way mux using a concurrent representation of SELECT statement
library IEEE; use IEEE.std_logic_1164.all; entity mux4 is port( a1 : in std_logic_vector(2 downto 0); a2 : in std_logic_vector(2 downto 0); a3 : in std_logic_vector(2 downto 0); a4 : in std_logic_vector(2 downto 0); sel : in std_logic_vector(1 downto 0); b : out std_logic_vector(2 downto 0)); end mux4; architecture rtl of mux4 is -- declarative part: empty begin with sel select b <= a1 when "00", a2 when "01", a3 when "10", a4 when others; end rtl;
MUX description using SELECT VHDL statement
Another VHDL description of a 4-way mux using a concurrent representation is given below
library IEEE; use IEEE.std_logic_1164.all; entity mux4 is port( a1 : in std_logic_vector(2 downto 0); a2 : in std_logic_vector(2 downto 0); a3 : in std_logic_vector(2 downto 0); a4 : in std_logic_vector(2 downto 0); sel : in std_logic_vector(1 downto 0); b : out std_logic_vector(2 downto 0)); end mux4; architecture rtl of mux4 is -- declarative part: empty begin b <= a1 when (sel = "00") else a2 when (sel = "01") else a3 when (sel = "10") else a4; end rtl;
MUX description using simple CONCURRENT VHDL statement
In Figure 2, Figure 3, Figure 4, are reported the implementation on Cyclone IV FPGA of the sequential and concurrent implementation of the VHDL code reported above. As clear, the circuit implementation is the same for both different VHDL coding style even if the RTL view can be different.
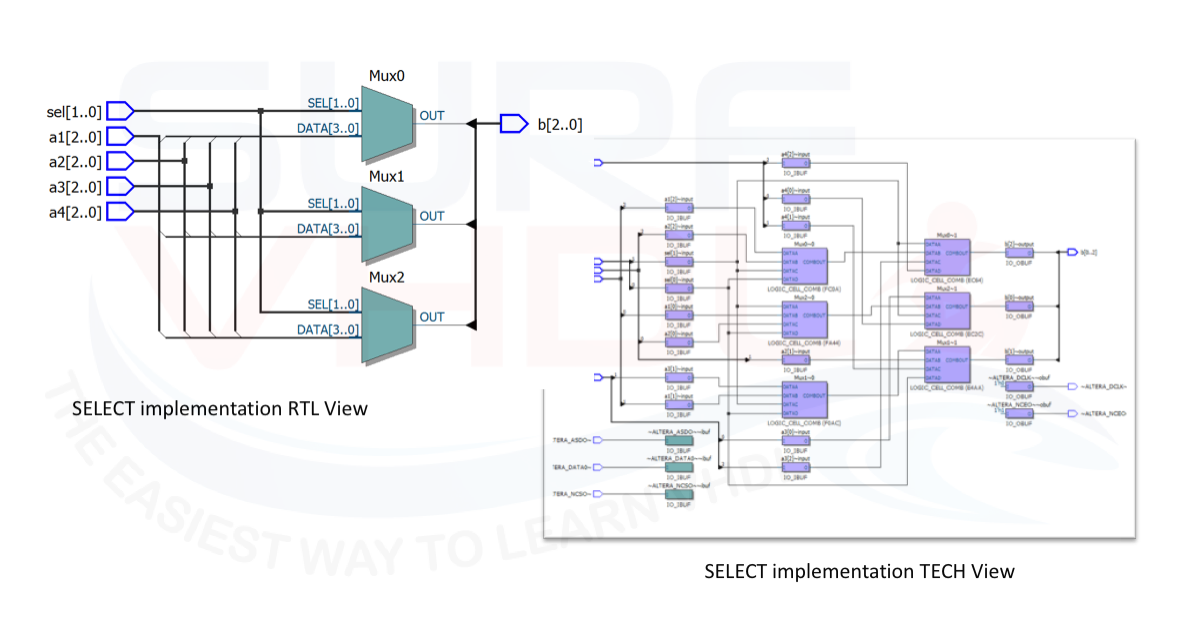
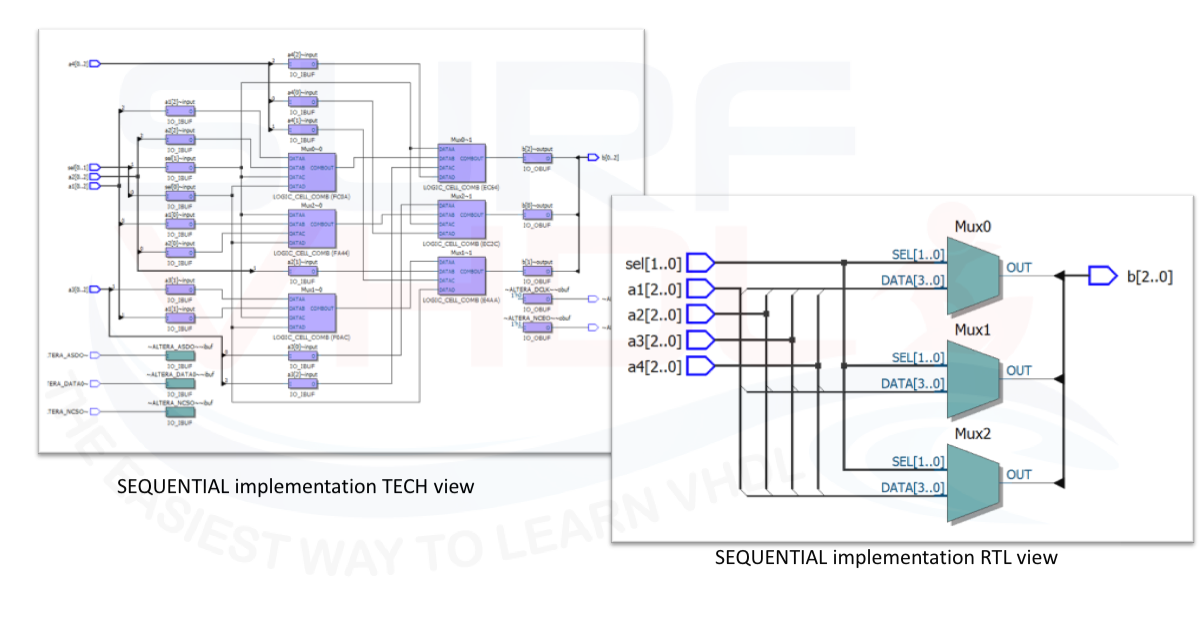
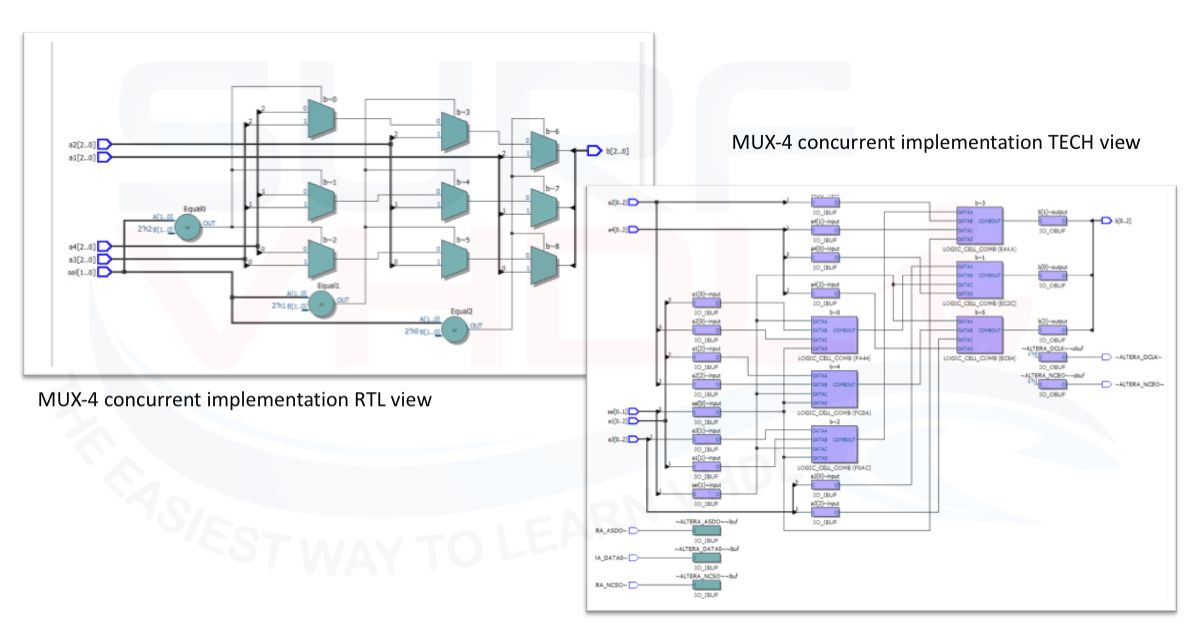
VHDL – MUX implementation using an array structure
If the number of the MUX input is a power of two, we can take advantage of the VHDL syntax, implementing the MUX in a very compact VHDL description.
To take advantage of the power of two number of input, we use the VHDL array structure.
In the VHDL code below, we define a user type that is an array of a signal using the same VHDL type of the MUX input.
The selector signal will be used as the index of the array.
The VHDL code is very compact and efficient as we can see below.
library ieee ; use ieee.std_logic_1164.all; use ieee.numeric_std.all; entity mux4 is port( d0 : in std_logic_vector(1 downto 0); d1 : in std_logic_vector(1 downto 0); d2 : in std_logic_vector(1 downto 0); d3 : in std_logic_vector(1 downto 0); s : in std_logic_vector(1 downto 0); m : out std_logic_vector(1 downto 0)); end mux4; architecture rtl of mux4 is type t_array_mux is array (0 to 3) of std_logic_vector(1 downto 0); signal array_mux : t_array_mux; begin array_mux(0) <= d0; array_mux(1) <= d1; array_mux(2) <= d2; array_mux(3) <= d3; m <= array_mux(to_integer(unsigned(s))); end rtl;
MUX description using simple ARRAY VHDL statement
Figure 6 reports the RTL and technology view of the MUX implementation using the array architecture implementation. As clear, the final implementation is totally equivalent to the concurrent or sequential one.
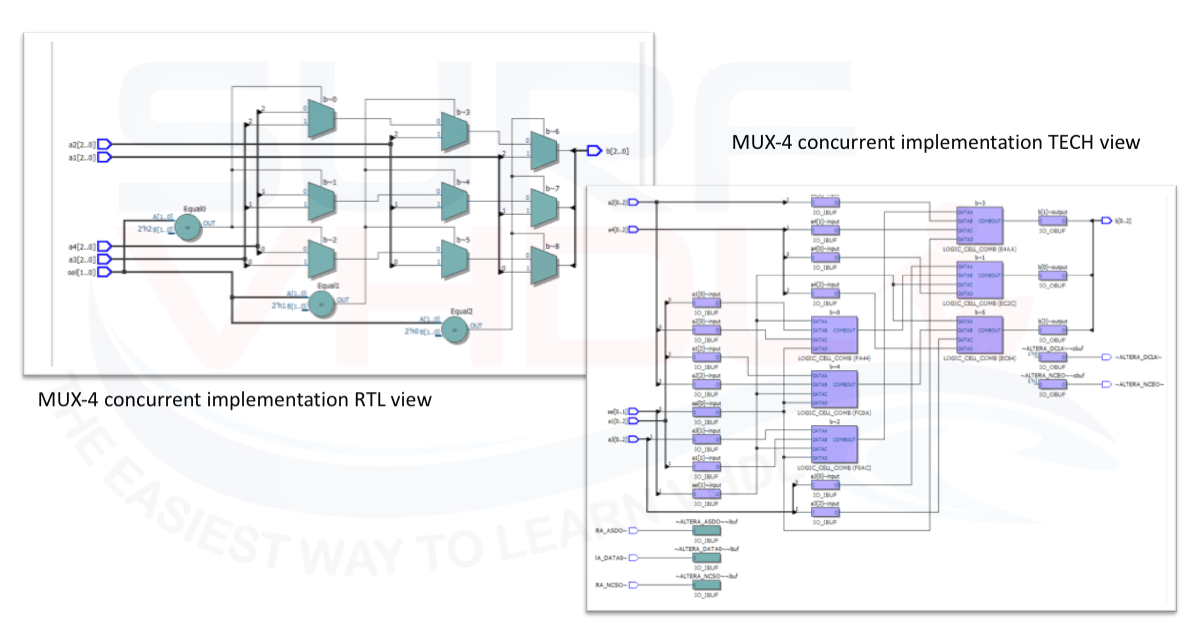
MUX VHDL Simulation
Figure 6 shows the MUX implementation. The MUX output the input value depending on the selector signal
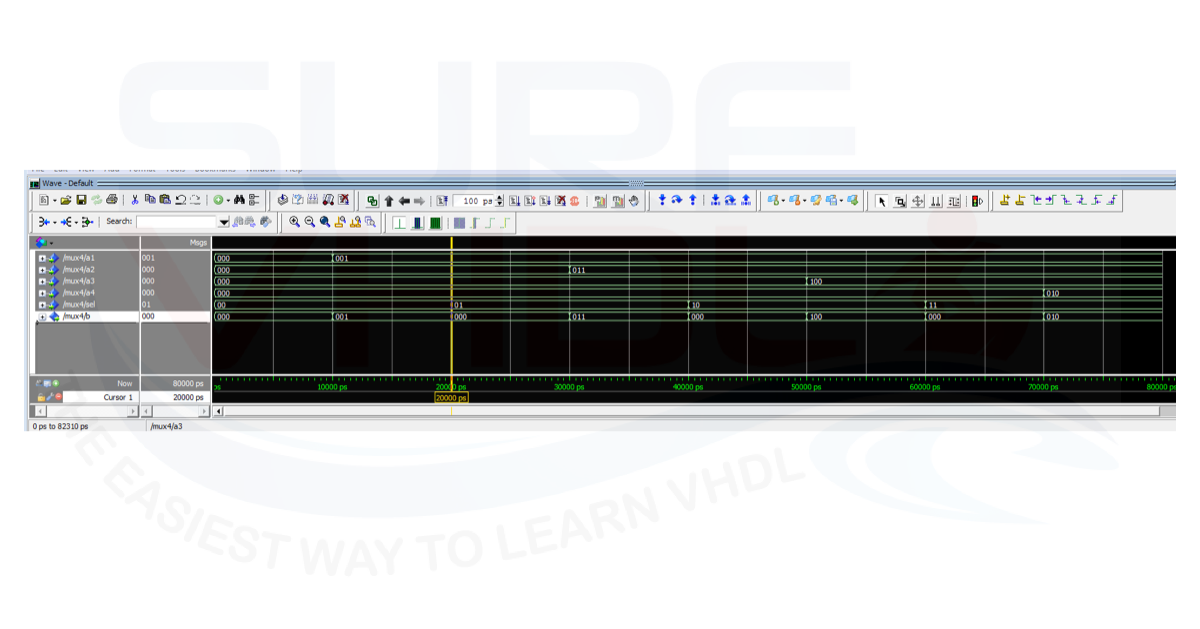
Conclusion
In this post, we addressed different ways to implement digital MUX in VHDL:
- VHDL Concurrent MUX implementation
- VHDL Sequential MUX implementation
- VHDL array based MUX implementation, when the input signals are a power of two
All the different VHDL descriptions are mapped into the same hardware.
References
[1] https://en.wikipedia.org/wiki/Multiplexer
[2] www.altera.com
[3] www.xilinx.com
[4] RTL HARDWARE DESIGN USING VHDL Coding for Efficiency, Portability, and Scalability – PONG P.CHU
Really good information, this information is excellent and essential for everyone. I am very very thankful to you for providing this kind of information.
Hoping to hear more about this stuff. Very effective and informative.