Serial Peripheral Interface Introduction
Before reading the post, if you need the VHDL code of the SPI controller, just put your email in the box you find in the post. There is no need to post a comment asking me for the code 🙂 If you don’t receive the email, please check your SPAM folder, enjoy!
The Serial Peripheral Interface (SPI) bus is a synchronous serial communication controller specification used for short-distance communication, primarily in embedded systems. The interface was developed by Motorola and has become a de-facto standard. Typical applications include sensors, Secure Digital cards, and liquid crystal displays.
SPI devices communicate in full-duplex mode using a master-slave architecture with a single master. The SPI master device originates from the frame for reading and writing. Multiple SPI slave devices are supported through selection with individual slave select (SS) lines as in Figure 2.
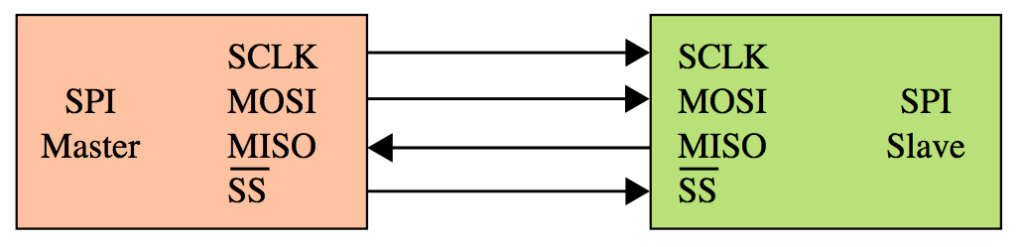
The SPI is a four-wire serial bus as you can see in Figure 1 and in Figure 2
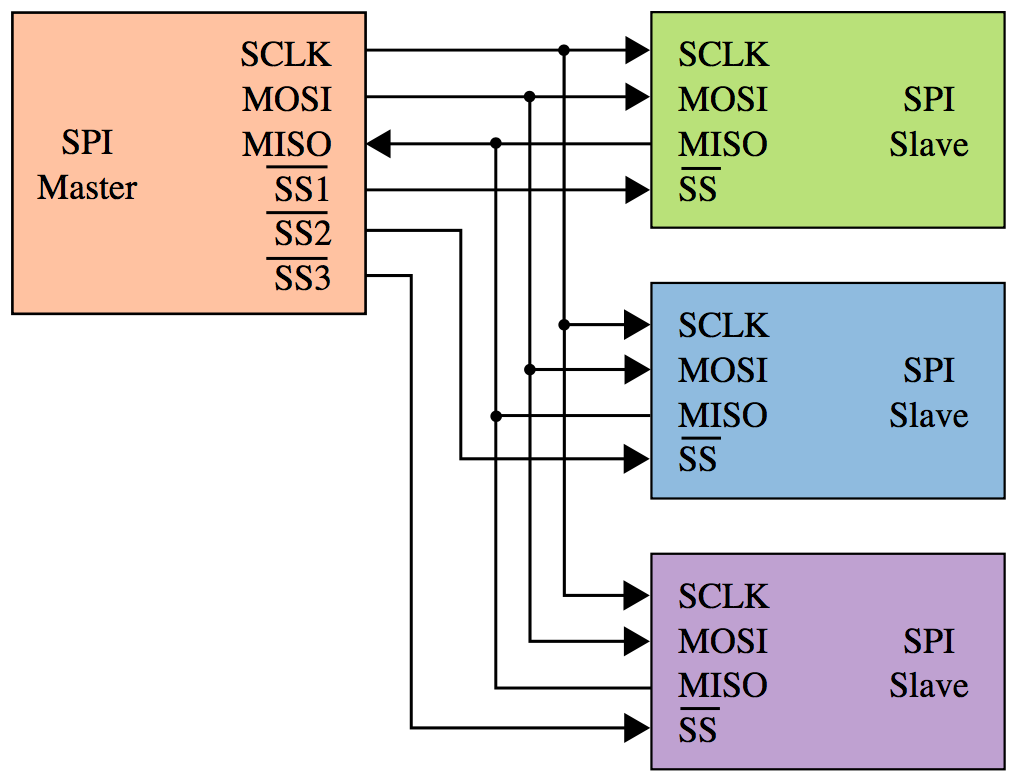
For further information see the Wikipedia Page dedicated to the SPI.
SPI controller architecture overview
The SPI bus specifies four logic signals:
- SCLK : Serial Clock (output from master).
- MOSI : Master Output, Slave Input (output from master).
- MISO : Master Input, Slave Output (output from slave).
- SS : Slave Select (active low, output from master).
You can find alternate naming, but the functionalities are the same.
SPI timing example is shown in Figure 4. The MOSI can be clocked either on the rising or falling edge of SCKL. If MISO change on the rising edge of SCLK, MISO will change on falling and vice versa.
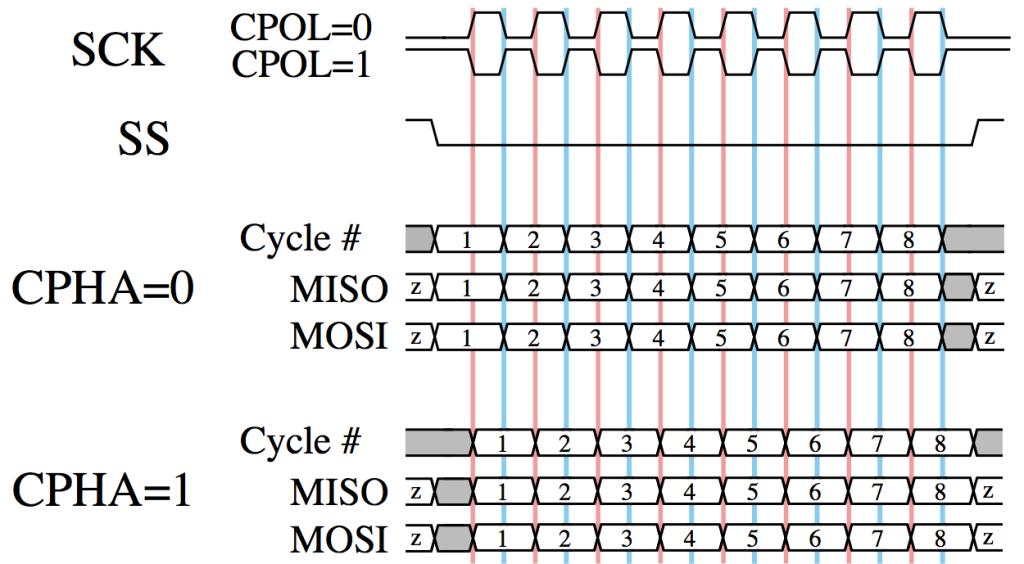
Data transmission begins on the falling edge of SS, then a number N of clock cycles will be provided. The MOSI is driven with the output data payload. The data payload can contain either data and command. If MOSI contains a command, i.e. read command, after a programmed number of SCLK cycles, MISO will be driven with the serial data value read from the slave.
SPI controller VHDL implementation
Before writing the SPI controller VHDL code, let’s review the SPI controller architecture of Figure 5
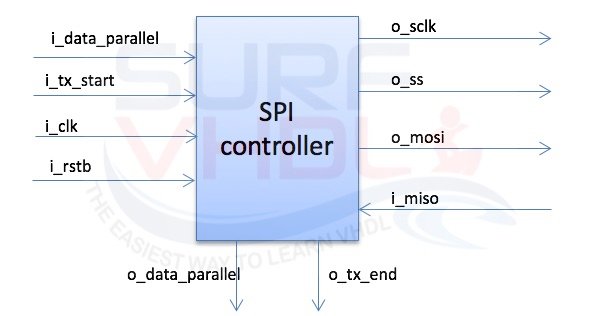
i_clk : input clock i_rstb : input power on reset active low i_tx_start : input start sending i_data_parallel on serial line o_tx_end : serial data sending terminated i_data_parallel : parallel data to sent o_data_parallel : parallel received data o_sclk : serial clock output o_ss : slave select o_mosi : serial data output i_miso : serial data input
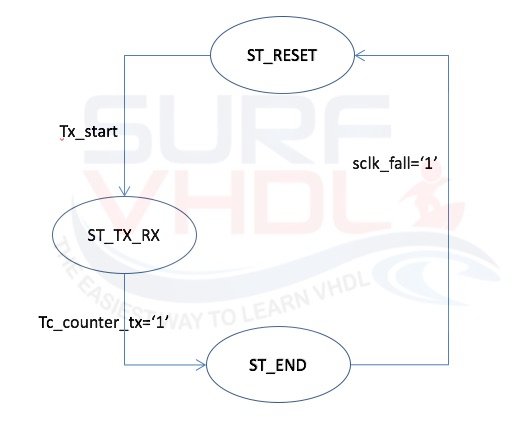
The SPI controller VHDL code will implement the FSM described in Figure 6. The input parallel data will be send using tx_start input signal. The FSM goes to “ST_TX_RX” state for a programmed number of clock cycles. During the data transmission, MISO input is sampled on the internal shift register. At the end of data transmission, the parallelized input is available on “o_parallel_data” output port and a pulse is generated on “o_tx_end” port. A possible VHDL implementation of SPI controller is available below:
library ieee; use ieee.std_logic_1164.all; use ieee.numeric_std.all; entity spi_controller is generic( N : integer := 8; -- number of bit to serialize CLK_DIV : integer := 100 ); -- input clock divider to generate output serial clock; o_sclk frequency = i_clk/(2*CLK_DIV) port ( i_clk : in std_logic; i_rstb : in std_logic; i_tx_start : in std_logic; -- start TX on serial line o_tx_end : out std_logic; -- TX data completed; o_data_parallel available i_data_parallel : in std_logic_vector(N-1 downto 0); -- data to sent o_data_parallel : out std_logic_vector(N-1 downto 0); -- received data o_sclk : out std_logic; o_ss : out std_logic; o_mosi : out std_logic; i_miso : in std_logic); end spi_controller; architecture rtl of spi_controller is type t_spi_controller_fsm is ( ST_RESET , ST_TX_RX , ST_END ); signal r_counter_clock : integer range 0 to CLK_DIV*2; signal r_sclk_rise : std_logic; signal r_sclk_fall : std_logic; signal r_counter_clock_ena : std_logic; signal r_counter_data : integer range 0 to N; signal w_tc_counter_data : std_logic; signal r_st_present : t_spi_controller_fsm; signal w_st_next : t_spi_controller_fsm; signal r_tx_start : std_logic; -- start TX on serial line signal r_tx_data : std_logic_vector(N-1 downto 0); -- data to sent signal r_rx_data : std_logic_vector(N-1 downto 0); -- received data begin w_tc_counter_data <= '0' when(r_counter_data>0) else '1'; -------------------------------------------------------------------- -- FSM p_state : process(i_clk,i_rstb) begin if(i_rstb='0') then r_st_present <= ST_RESET; elsif(rising_edge(i_clk)) then r_st_present <= w_st_next; end if; end process p_state; p_comb : process( r_st_present , w_tc_counter_data , r_tx_start , r_sclk_rise , r_sclk_fall ) begin case r_st_present is when ST_TX_RX => if (w_tc_counter_data='1') and (r_sclk_rise='1') then w_st_next <= ST_END ; else w_st_next <= ST_TX_RX ; end if; when ST_END => if(r_sclk_fall='1') then w_st_next <= ST_RESET ; else w_st_next <= ST_END ; end if; when others => -- ST_RESET if(r_tx_start='1') then w_st_next <= ST_TX_RX ; else w_st_next <= ST_RESET ; end if; end case; end process p_comb; p_state_out : process(i_clk,i_rstb) begin if(i_rstb='0') then r_tx_start <= '0'; r_tx_data <= (others=>'0'); r_rx_data <= (others=>'0'); o_tx_end <= '0'; o_data_parallel <= (others=>'0'); r_counter_data <= N-1; r_counter_clock_ena <= '0'; o_sclk <= '1'; o_ss <= '1'; o_mosi <= '1'; elsif(rising_edge(i_clk)) then r_tx_start <= i_tx_start; case r_st_present is when ST_TX_RX => o_tx_end <= '0'; r_counter_clock_ena <= '1'; if(r_sclk_rise='1') then o_sclk <= '1'; r_rx_data <= r_rx_data(N-2 downto 0)&i_miso; if(r_counter_data>0) then r_counter_data <= r_counter_data - 1; end if; elsif(r_sclk_fall='1') then o_sclk <= '0'; o_mosi <= r_tx_data(N-1); r_tx_data <= r_tx_data(N-2 downto 0)&'1'; end if; o_ss <= '0'; when ST_END => o_tx_end <= r_sclk_fall; o_data_parallel <= r_rx_data; r_counter_data <= N-1; r_counter_clock_ena <= '1'; o_ss <= '0'; when others => -- ST_RESET r_tx_data <= i_data_parallel; o_tx_end <= '0'; r_counter_data <= N-1; r_counter_clock_ena <= '0'; o_sclk <= '1'; o_ss <= '1'; o_mosi <= '1'; end case; end if; end process p_state_out; p_counter_clock : process(i_clk,i_rstb) begin if(i_rstb='0') then r_counter_clock <= 0; r_sclk_rise <= '0'; r_sclk_fall <= '0'; elsif(rising_edge(i_clk)) then if(r_counter_clock_ena='1') then -- sclk = '1' by default if(r_counter_clock=CLK_DIV-1) then -- firse edge = fall r_counter_clock <= r_counter_clock + 1; r_sclk_rise <= '0'; r_sclk_fall <= '1'; elsif(r_counter_clock=(CLK_DIV*2)-1) then r_counter_clock <= 0; r_sclk_rise <= '1'; r_sclk_fall <= '0'; else r_counter_clock <= r_counter_clock + 1; r_sclk_rise <= '0'; r_sclk_fall <= '0'; end if; else r_counter_clock <= 0; r_sclk_rise <= '0'; r_sclk_fall <= '0'; end if; end if; end process p_counter_clock; end rtl;
In the following figures, there are three examples of SPI protocol simulation. In the Modelsim windows is clear the SPI protocol behavior: input parallel data is serialized on MOSI output port. The MISO input data is parallelized in the o_parallel_data port of the SPI controller.
Figure 7 shows an overall simulation view of three SPI cycles
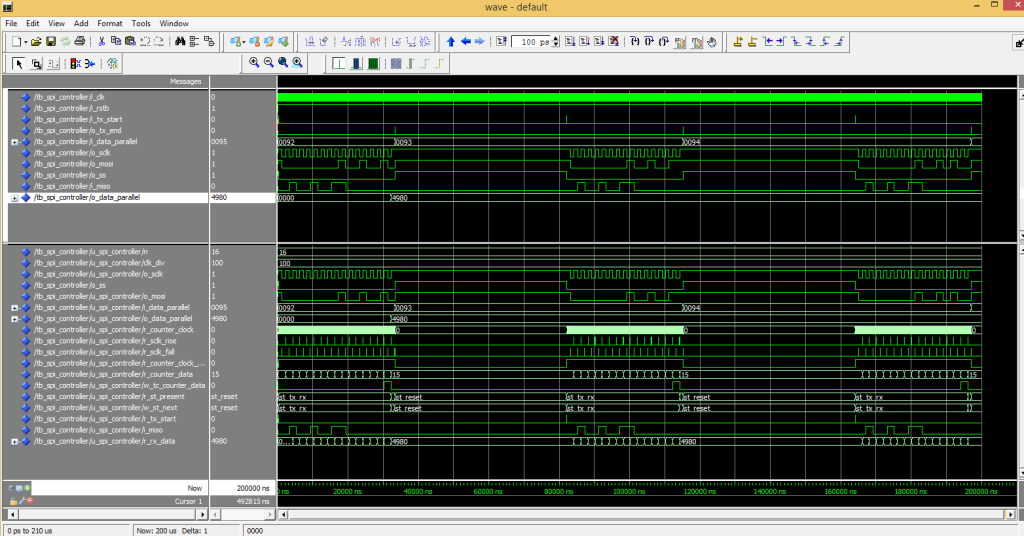
Figure 8 shows a zoom on the second SPI cycle
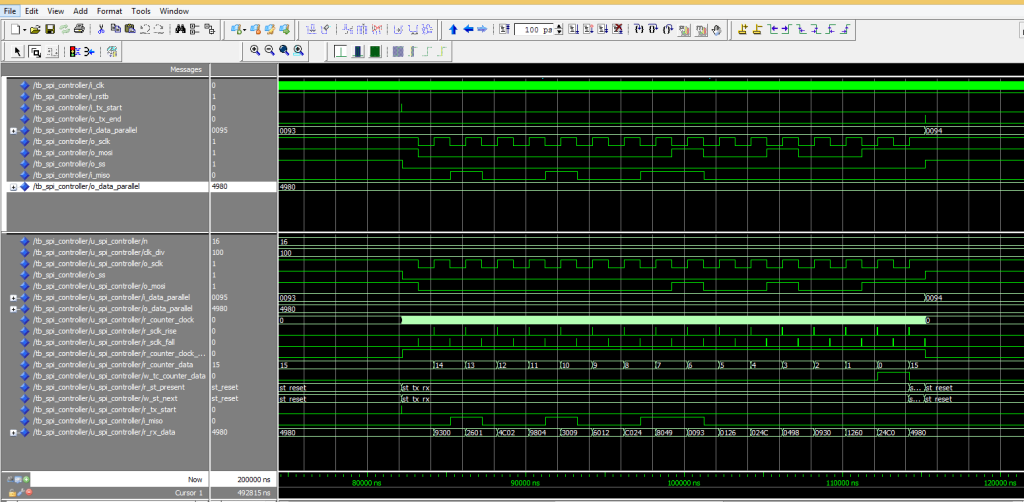
Figure 9 shows a zoom on the SPI cycle start. The system clock is set to 10 ns in the simulation. The SCLK is generated dividing by 200 the system clock: 100 for high phase, 100 for low phase as specified in the generic “CLK_DIV”
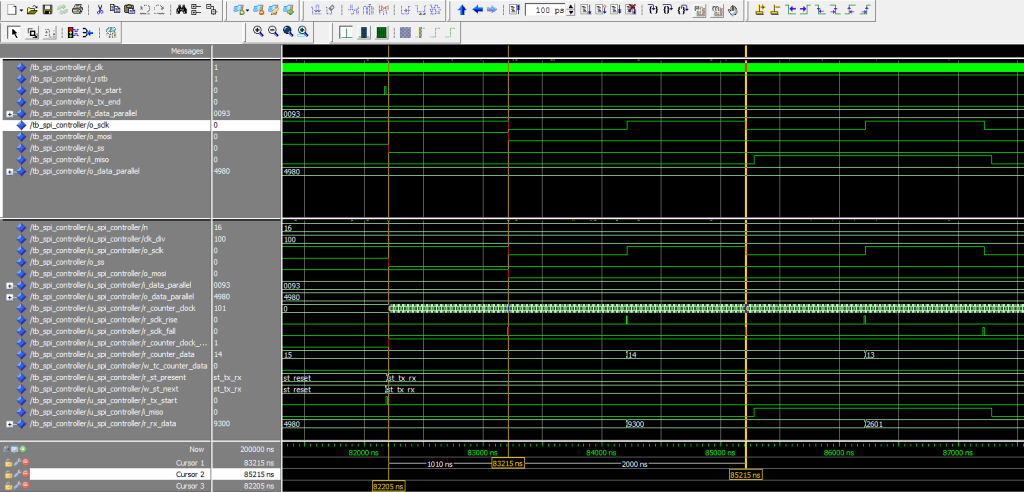
The SPI controller VHDL code above is technology independent and can be implemented either on FPGA or ASIC.
Figure 10 shows Altera Quartus II RTL viewer of the SPI VHDL code implementation above.
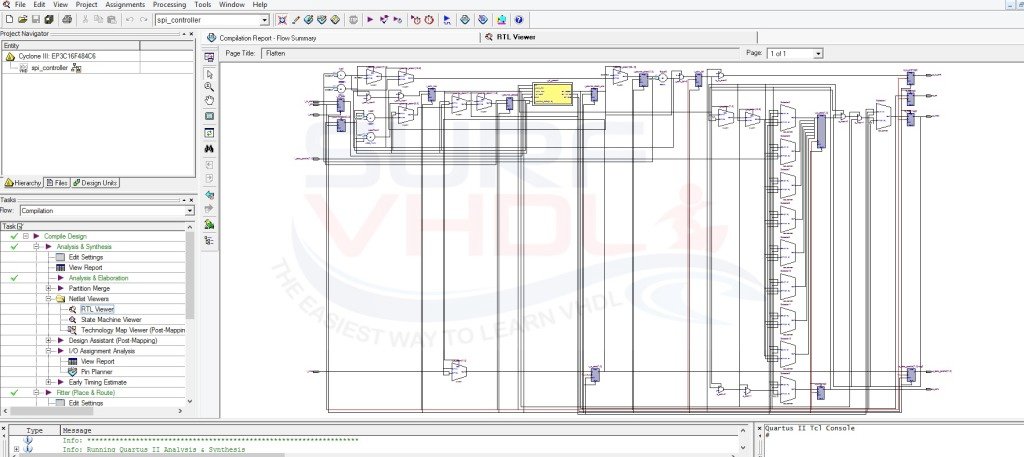
The SPI controller VHDL code has been tested on Altera Cyclone III FPGA with 8 bit-serial and parallel data.
The implementation takes 58 Logic Element (LE) and performs @ 400 MHz as reported in the Quartus II area report and timing report below.
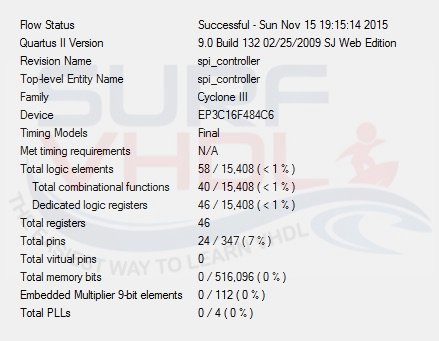

References:
https://en.wikipedia.org/wiki/Serial_Peripheral_Interface_Bus
If you appreciated this post, please help me to share it with your friend.
[social_sharing style=”style-7″ fb_like_url=”https://surf-vhdl.com/how-to-design-spi-controller-in-vhdl” fb_color=”light” fb_lang=”en_US” fb_text=”like” fb_button_text=”Share” tw_lang=”en” tw_url=”https://surf-vhdl.com/how-to-design-spi-controller-in-vhdl” tw_button_text=”Share” g_url=”https://surf-vhdl.com/how-to-design-spi-controller-in-vhdl” g_lang=”en-GB” g_button_text=”Share” linkedin_url=”https://surf-vhdl.com/how-to-design-spi-controller-in-vhdl” linkedin_lang=”en_US” alignment=”center”]
If you need to contact me, please write to: surf.vhdl@gmail.com
I appreciate any of your comment, please post below:
Thanks for the great code.
I wonder which SPI mode is used (CPOL and CPHA)? and can all the modes be added easily?
Thanks,
Meto
just great
Thank you!
This is fantastic, do you happen to have line comments for this code?
thank you
The code is it’s own best comment. Figure it out.
Pls share SPI interface code with testbench
hello,
how to convert the analog signals in the form of text file to digital?
what signal do you want to convert?
I don’t understand the question
heyy do you have a vhdl testbench for this plz ?
send me a mail
please, send me and email so I will reply
Hello,
thanks for the tutorial. Can you send me the testbench code for this spi master please.
Hello Dear,
do you have any idea how FPGA read data from DSP and microcontroller and vice versa, means write to DSP and microcontroller?
Appreciate your answer
generally, the micro is the master of the communication and the FPGA is a peripheral for the micro.
take a look here
http://surf-vhdl.com/how-to-interface-a-fpga-processor-with-vhdl-peripheral/
Can you send i2c vhdl code
Could you please send me a testbench for this?
send me an email
sorry, I forgot.
yunlongqi@my.unt.edu
Thank you.
check your inbox
Please send me SPI testbench Catanat1717@gmail.com
hi did you receive the test bench?
aparajita.neha@gmail.com
i send the mail but i didn’t receive test bench
please, check your spam or promotion folder, sometimes gmail put the mail there
Ciao
Can I have the test bench for the above spi-controller. Thank you
just put your email in the box above to receive all the test bench
cheers!
Hey!
Could I maybe use the test bench as well please?
cucuji4081@gmail.com
can you send me a testbench of this spi controller, thanks
(this is by far I found the most logical spi controller in VHDL out there.)
can you send me a vhdl testbench spi plz?
my email: imax16041998@gmail.com
please send me test bench for the above code
email_id : basavarajjamboti@gmail.com
can you send me the testbench please for slave spi
hello did you find the test bench? I need it too.
hey can u send me the testbench if possible, my email is fikrixzx@gmail.com
check your inbox
cheers
could you please send me the test bench for this sathishmitta1306@gmail.com
Hello
Can you send me testbench 🙂 ?
rayjohn8901@gmail.com
Thank you ! 🙂
send me an email
pls can you send me the test bench code. thanks. patrickonen@gmail.com
send me an email
rayjohn8901@gmail.com
check your inbox
hello please send me the test bench
thanks
Hello.
Could you please send me testbench 🙂 ?
Hii Thanks for the Info!
Please send me the testbench for the code .
email: abhishekgoswami610@gmail.com
check your inbox
Pls could you send me the test bench code. Thanks
you can download from the post just insert you e-mail
Hello
Can you send me testbench 🙂 ?
gf.diluca@gmail.com
Thank you ! 🙂
just use the form to get the VHDL code
can you please send me the testbench
somesh.rk73@gmail.com
just download it from the form in the post
pls can you send me the test bench code. thanks
sadeghimarasht@gmail.com
just put your email in the box inside the post and you will receive the code
HI, I have a question :this spi code include master an slave? Thanks.
Hi, i want to buy the de0 nano soc for RF application.
https://media.digikey.com/pdf/Data%20Sheets/Terasic%20Technologies/DE0-Nano-SoC_UM.pdf
I have to use SPI and i am going to see your code. Do you think it is possible for me to use it to control a Tuner (Tuner is the slave and FPGA is the master) ? If yes, do you have a testbench ?
My email : simonleo33@gmail.com
Cordially and thanks for your attention.
You should check the datasheet of the tuner, but I think yes.
In any case, you can modify the code to easily adapt it to your device.
You can download the test bench directly from this page,
just insert your email address in the box inside the post
Hello
Can you send me testbench 🙂 ?
prashantfunde91@gmail.com
Thank you ! 🙂
Hello
Can you send me testbench 🙂 ?
prasantfunde91@gmail.com
Thank you ! 🙂
just input your email in the BOX in the post and you will receive the files
Cheers!
Hello,
can you send me slave spi code and test bench?
Thanks.
just put your email in the box above and you will receive the code
cheers!
Hello,
can you send me (master and slave spi) code and test bench?
Thanks.
sadeghimarasht@gmail.com
Hello,
can you send me (master and slave spi) code and test bench?
Thanks.
sadeghimarasht@gmail.com
Please do send me the testbench…My Mail-id umeshcsjuly@gmail.com
Thanks
just put your email in the box above and you will get it in your mailbox
can u give me the testbench to this ? email is muhd27hafeez@gmail.com
just put your email in the box above
Cheers
hello this pleaseee send me the test bench I need it
donyakhaledyan@yahoo.com
Thanks for your great article.
Please send me the SPI testbench, via anzun.guen@gmail.com
Thanks a lot.
just put your email in the box above and you will receive all the files in your mailbox
Cheers!
can u send me the testbench if possible, my email is nguyenthanhsonht2005@gmail.com
just put your email in the box above
can u send me the testbench
just put your email in the box and you will receive the VHDL code
Hello,
can you send me (master and slave spi) code and test bench?
Thanks.
anurkapisiz@gmail.com
just put your email in the box above
Thanks,
Could you please provide me the testbench?
just put your email in the box above
Regards
I am not able to understand the signal ports meaning.
what do you not understand?
Hello 🙂
Can you send me code and testbench? (airspacemonkey@gmail.com)
Thank you!
just sign in using the box above
cheers
Hallo,
please send me the testbench. Thanks
just put your email in the box above
will you please provide me the testbench for this code
just put your email in the box above
just put your email in the box above
This is a really great website. Thank you for all the material you have made available here. I’m hoping to be able to move working away from Microcontrollers to fpga’s in the near future!!
Can you please provide the test bench ? email : rajrocks146@gmail.com
you can download it from this page, just put your email in the box above
can u send me the testbench
just download from this page
Hi, I would need test bench files. Thanks
just insert your email in the box above in the post
I’m interested in the SPI testbench – I put my name and email in the box above as suggested, but it hasn’t provided access to it. I don;t see a link on this page, and searching for “testbench” yields no results. Can you send a link?
maybe the email is in the SPAM folder.
I resent it
ciao
Hi,
Could you please send the test bench file?Thanks
setatcicek@gmail.com
if you put your email in the box above you will receive the test bench.
Sometimes the email goes in the SPAM folder, please check there
ciao
hi
could you please send the test bench file.thank you
kurichetimounika@gmail.com
Hi mounika, you should have been received. Please check the spam folder.
ciao
Hi
could you send the test bench file? thank you.
aliguul1@gmail.com
Please, put your email in the box and if you didn’t receive anything check the spam folder
Hello! Could yoou please send me a testbench, the box below doesn”t work correctly(
rada.belz@yandex.ru
Thank you very much!
If you didn’t receive anything, please check the spam folder
Hi,
Could you please send me the testbench file? (el_miniparra@hotmail.com)
Many Thanks
Please, put your email in the box and if you didn’t receive anything check the spam folder
email: el_miniparra@hotmail.com
I’ve not received your email.
Thanks
please, check your spam folder and let me know
ciao
Hello, could you please send me the test bench?
donyakhaledyan@yahoo.com
hi,
just put your email in the box and you will receive it.
ciao
Hello! Could you please send the testbench to me? This box above doesn’t work actually(
rada.belz@yandex.ru
Thank you very much!
Best regards,
Alena
hi
could you please send the test bench file?
thank you
el_miniparra@hotmail.com
hello I need the test bench too.do you have that? I will be thanks of you if you send me that.this is my email
donyakhaledyan@yahoo.com
Hi, just put your email in the box that appears in the post windows.
Let me know if you have a problem
thanks for sharing. Sir can you plz send me the SPI master-slave code with testbench. I need it for learning. Thanks in advance.
Hi, put your email in the box you find in the post.
If you do not receive anything, please check your spam folder
Ciao!
hi, can you please send me the testbench too?
thank you
put your email in the box you find on this page and you will find it in your inbox.
If you did it, please check your spam folder.
Ciao
Happy new year!
hello, I didn’t receive the test bench. if it’s possible please send me again
if you didn’t get the files, please check your spam or promotion folder
ciao
Could you please send me a testbench for this?
donyakhaledyan@yahoo.com
Hello,
Do you have the controller from the Slave point of view? I need a SPI Slave controller.
Thanks
Not by now, in the future will be available
hi,
just put your email in the box and you will receive it.
ciao
I am RF student but have found writing VHDL code to represent the hardware circuits I have work
with, only the simple circuits to date, and running the simulation is great.
Your SPI code that will take me time to follow.
My next step is to run the simulation
Could you provide the test bench code
just put your email in the box above and you will get the code. If you already do this, check your spam folder
ciao
Hi, I need the testbench , thanks for your great job!
duzhenhuan@gmail.com
just put your email in the box above.
If you don’t get the code check in the spam or promotion folder
it’s a very good post. currently, I am working on this, would you please send the project files on deepakkumar7805@gmail.com
just put your email in the box and you will get it in your mailbox
Hi
Can you please share the testbench for spi controller? Thanks very much My email address is paula179179@gmail.com
just put your email in the box and you will get it in your mailbox
can you please provide me the code?
put your email in the box above
ciao
Plz send test bench
put your email in the box above.
you can also listen to my podcast
https://itunes.apple.com/it/podcast/qa-3-plzz-send-the-test-bench/id1449367271?i=1000429261648&l=en&mt=2
What if i have to support “N” slaves? for example 3 slave i need to support using a single master
But i have designed a SPI master for single slave. I dont want to just instantiate and connect to 3 slaves…
What is the solution?
I dont see any forums explaining about supporting multiple slave (in the code) … pls reply it will be helpful
you can use multiple CS
please share your testbench
Can you please send me test bench on girija.bhagwat@gmail.com?
Hi,
can u send me the testbench
Thanks
just put your email in the box above
Can I get a copy of the test bench please?
jannyliao@hotmail.com
Thank you!
put your email in the box above and will get the code directly in your mailbox
Ciao
if my data is “010101010101010101010101010101010” then
the how to get the out for the given data as
if either 0 or 1 is there…
i want to full cycle for either 0 or 1…
how to get that?
plsss…..spport me …
what do you mean with “full cycle”?
Can I get a copy of the test bench please?
e.ulucan@mekoptronik.com.tr
Thank you…
Can I get a copy of the test bench please?
e.ulucan@mekoptronik.com.tr
Thank you!
Hi,
can u send me the testbench
e.ulucan@mekoptronik.com.tr
Thanks
Hi,
can you send me the testbench please?
anna.late87@gmail.com
Thank you!
Could you send me the testbench files, please?
just put your email in the box above and you will get it in your mailbox
somebody can send me testbench now? i verry need that now….
my email: imax16041998@gmail.com
p/s: i confirm email but can’t see any testbench to download?
hello! Send me testbench plzzzzzz, i need that
i confirm email but not see testbench anywhere?
did you get it?
if not check your spam or promotion folder
please share details on oolulalbaab@gmail.com
which details?
Hi, surf!
How to implement a continuous mode in this code?
Thanks!
just generate the start pulse every N clock cycle, where N is the word length
Hi,
can you send me the testbench please?
sadat_s72@gmx.de
Thank you!
Can you send me the testbench,please?
Can you send me the testbench,please?
my mail : sarmusak.burak@gmail.com
i need help on vhdl master and arduino slave spi
thanks
Please send me SPI testbench
sumer1_2006@yahoo.com
put your email in the box on the right
Hi, can you send me the testbench please?
my email : ryanklkinsella@gmail.com
Thank you!
Hi could you please send me the testbench?
Email: dagr8one111@gmail.com
register in the box on the rigth
can u send the testbench for this
karth252@gmail.com
Just put your email in the box on the right
Just put your email in the box on the right
spi vhdl testbench,please
spi vhdl testbench,please
hey can you send me the testbench. thank you, logical code!
jthurysz@gmail.com
Just put your email in the box on the right
Hi could you please send me the testbench, please?
Email: ydmoon72@gmail.com
I hope you get it. thanks.
Just put your email in the box on the right
can i have your testbench if you don’t mind.
thank you!
caotuanhung396@gmail.com
please send me a test bench for the above code. It would be of great help.
email: nitin09295@gmail.com
Can you also please help me with the knowledge of all the registers we have used to deploy this design as well. I am in great need.
Thanks and Regards
nitin09295@gmail.com
They are documented in the post. What are your doubts?
Hi could you please send me the testbench, please?
mail: akkurtcagatay@gmail.com
Can you send the .vht, the prompt doesn’t work, it didn’t send me the testbench, please? 🙁
The box to the right did not work. Will you please send me the testbench? Thanks!
check your spam folder
hey can u send me the testbench if possible, my email is kingmev98@gmail.com
hello bro i want to make a transmitter for my drone ,this spi controller can works ?
I don’t know, depends on what you need
Hi could you send me the testbench ?
and you have some experience with SPI to falsh memory?
Email: mhv005@post.uit.no
Greetings,
Can you please share the test bench code? Thank you!
gil.gonzalez.navarro90@gmail.com
can you please upload a practical video of this code ?
Sir could you please send me Test bench code
mprakash.2405@gmail.com
thank you so much
Awesome post.. can you please share TestBench @ piasquare@gmail.com
Sir could you please send me Test bench code
kcimhajar@gmail.com
thank you so much
Hi, can you please share me the testbench for the SPI controller?
Here my email: penguinlove1022@gmail.com
Thank you.
Can you send me the testbench,please?
my mail : liran09@gmail.com
I think this is the form to receive a copy of the testbench?
yes
Can you send me the spi slave code, please?
Mail: anibal.tapia.97@hotmail.com
Can you send me the testbench,please?
my mail : mohammadnorouzy@gmail.com
Can you send me the testbench, please?
my mail : izmirm@itu.edu.tr
Thanks for advance.
I need the VHDL code for SPI controller (master)
HELLO
Can you send me VHDL code how to use /assign frequency to input Clk in VHDL using PLL ip core
it depends on the PLL
Hi,
Thanks for the tutorial. Can you send me the testbench code for this spi master please.
Can you send me the testbench and the code for W5500 LAN module if you can please.
mail: austaleke@yahoo.com
Thanks in advance
hello,
can u also send me the testbence.vhd?
shixiangxue.de@gmail.com
thanks in regard,
Shixiang
Hi,
could you please send me the test bench?
I put my email in the box but I received nothing.
my email: nargesiexo@gmail.com
Hello sir,
Can you please send me the test bench..
phalrnd@gmail.com
Hello, can you please send me the VHDL RTL for a SPI slave? Thanks.
John
j.petrik@hotmail.com
I need VHDL SPI code
Hello,I want VHDL SPI code,thanks.
Hi, can you please send me the VHDL RTL for a SPI slave? Thanks.
I need VHDL SPI code
Thanks a lot!
can you please share the testbench code for this master spi .
Can you send me the testbench,please?
my mail : zahrasad1616@gmail.com
Can you send me the test bench, please?
Thank you
jenkinswill935@gmail.com
Can you send me code and testbench please.
Thanks
Thank you. Could you share the test bench code
904692309@qq.com, Pls send me the RTL and test bench, thanks a lot!
Can I have the VHDL test bench for the above spi-controller.
Thank you
email: shmuel.assiag2@gmail.com